While refactoring the URL routing tree of a web project, I performed some changes in the route paths and noticed that I was being redirected, so then I remembered that I had redirection rules applied (which, in NextJs, are set on the next.config.js
, which I personally find inconvenient). Because I didn’t need those redirect rules anymore, I removed them and restarted the web server. But the redirections were still happening.
I am embarrassed of saying how much time I spent debugging this, somewhy advocating this behavior to the changes in the route paths I’ve made, given that I have deleted all the redirect config. Because of that bias, I didn’t consider what now seems obvious, which thankfully and finally I understood: it was a redirection configuration (not a change in the route paths).
I went back to my next.config.js
and looked at the diff of what I’ve removed, and there it was , a big note that read: “I’m dumb lol”. The redirect configuration that I removed was setting permanent redirects instead of temporary redirect, HTTP codes 301 and 307 respectively.
Now, this was not incorrect, I indeed wanted to redirect permanently from one route to the other, the ‘permanent’ setting was applied on purpose and explicitly, but when I removed them, I forgot this part: 301 Permanent Redirect is cached in the browser. So, even if I’d removed the redirect configuration, the browser was using it’s own cached version.
Example
https://www.youtube.com/watch?v=loia-RbdPKA
Code of the video: https://github.com/frnkq/blog-code-examples/tree/main/mind-the-cache
As it can be seen in the video, I:
- Start with a basic HTTP server running with two routes: /home & /pageB
- Redirect /home to /pageB
- Navigate to /home on browser
- I am being redirected to /pageB
- Remove the redirect
- Navigate to /home on browser
- I am are redirected to pageB
And even if I clear cookies, the redirect is still there. For clearing this ‘redirect cache’, you would have to remove the site from your web history, which I find odd, shouldn’t be this part of site data instead of the site history? It doesn’t even seem to be an official documentation for this, MDN Web Docs: Redirection. You can ignore this behavior if you disable cache, or can also set cache rules using the Cache-Control header.
For the video I used NextJs & React because it was the stack I was using when I learned about this, but a much more simple example can be achieved with PHP:
//index.php
<?php
echo "Hello World from /index.php";
/* header('Location: /pageB.php', true, 301); */
//pageB.php
<?php
echo "Hello world from /pageB";
If you start a PHP server (with php -S localhost:80
) and open it with the browser, you’ll see “Hello World from /index.php”. Now, stop the browser and uncomment the second line of the index.php, run and access the server again, you’ll be redirected to /pageB.php and you’ll see “Hello world from /pageB”. Now comment again the second line of the index.php, re-run the server and you’ll see that you are still being redirected to /pageB.
So…if you are working with redirects:
- Have in mind that your browser may be caching redirects
- Remember the whole spectrum of 300 status codes
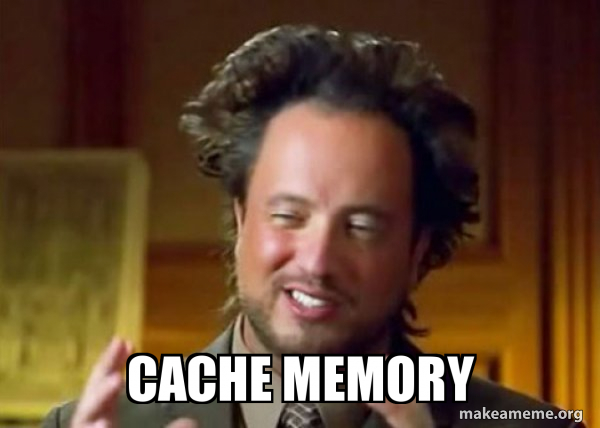